Authentication with Access Tokens
FastPix has a robust authentication mechanism for its API requests, ensuring secure communication between clients and its API through Access Tokens. These tokens serve as the gateway to the API, granting authorized access to various endpoints. Here’s a more detailed breakdown:
Access token permissions
- Read and Write Permissions:
If you need to create and modify resources (e.g., media, direct uploads, live streams), ensure your Access Token has both read and write permissions. - Read-Only Permissions:
If your code primarily involvesGET
requests without creating resources, limit the Access Token to read-only permissions.

Workspace-specific tokens
Access tokens are workspace-specific. For example:
- A development token is meant for testing and experimentation and is not valid for requests in a production workspace.
- A production token is valid for live requests in a production environment and similarly can’t be used for a development workspace.
When generating an Access Token, ensure it aligns with your intended use case. Mixing up tokens could lead to unexpected results!
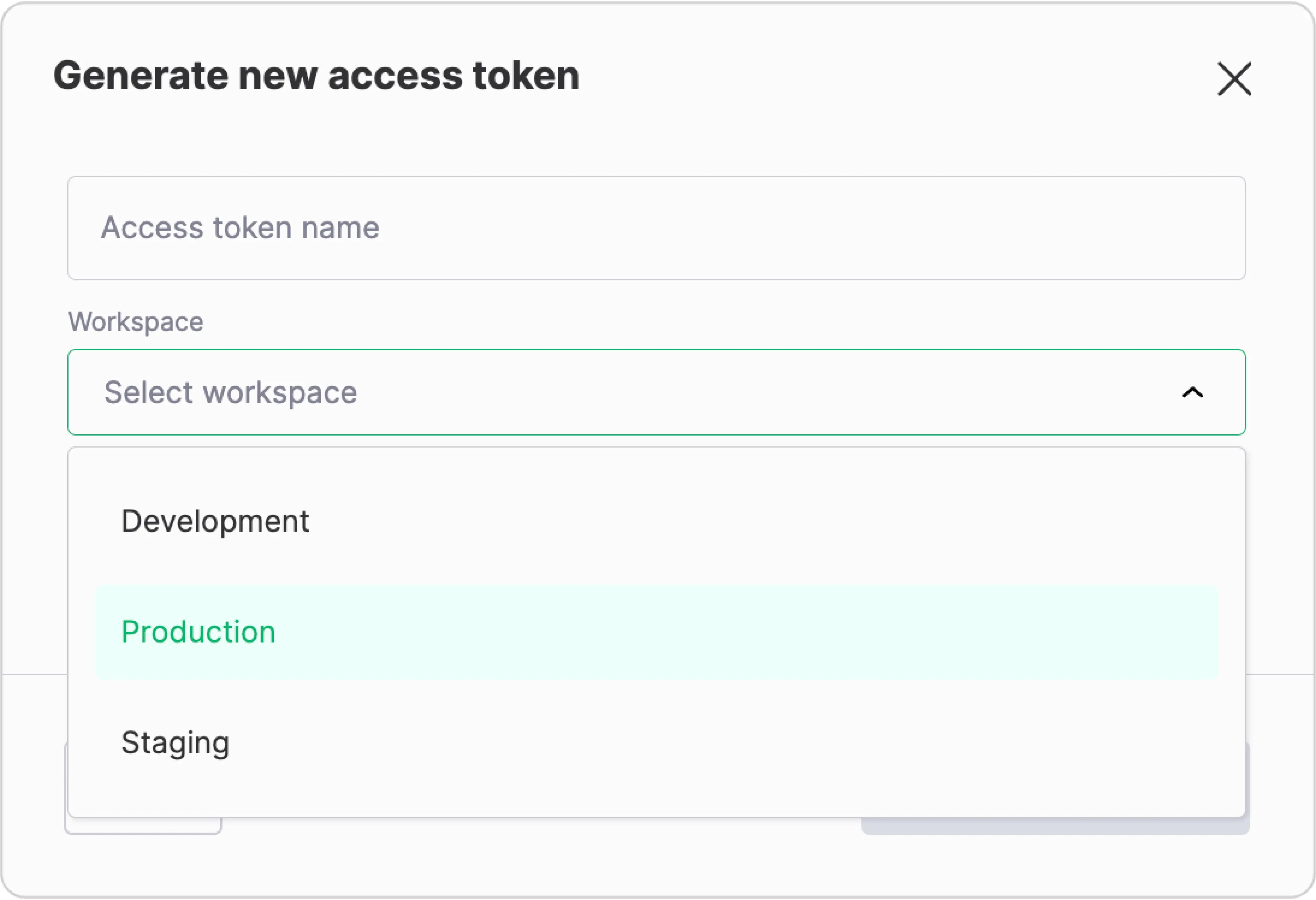
Access token components
Each API request made to FastPix must include an Access Token, which consists of two critical components:
- Access Token ID: The Access Token’s ID acts as the username. It uniquely identifies the client or application making the request.
- Secret Key: The secret key functions as the password. It’s a sensitive piece of information that must be kept confidential.
Handling the secret key
- FastPix takes security seriously. Instead of storing the secret key directly, it maintains a secure hash of the key. This approach ensures that even if the hash is compromised, the original secret remains hidden.
- However, this means that FastPix cannot recover a lost secret key. If it’s misplaced, the only solution is to create a new Access Token.
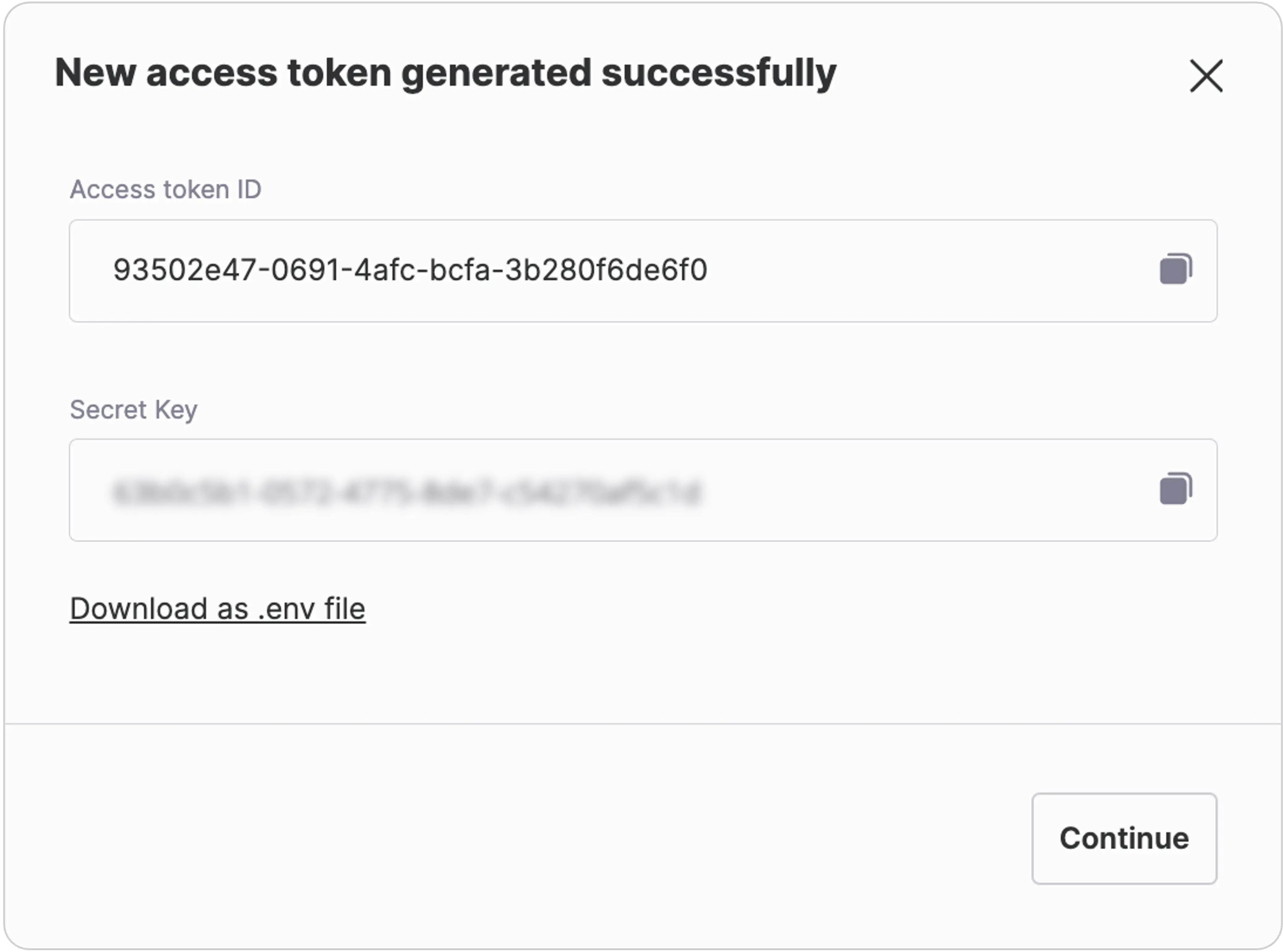
PLEASE NOTE
To keep the Access Token information (Secret Key) for future use, download an workspace file (.env) that stores these details. FastPix doesn’t store the exact Secret Key.
Revoke access token
In the unfortunate event of a leaked secret key (due to a security breach or accidental exposure), immediate action is necessary. Revoke the corresponding Access Token promptly. You can do this from the Org Settings page: Access Tokens. By revoking the token, you prevent unauthorized access and maintain the integrity of your FastPix account.
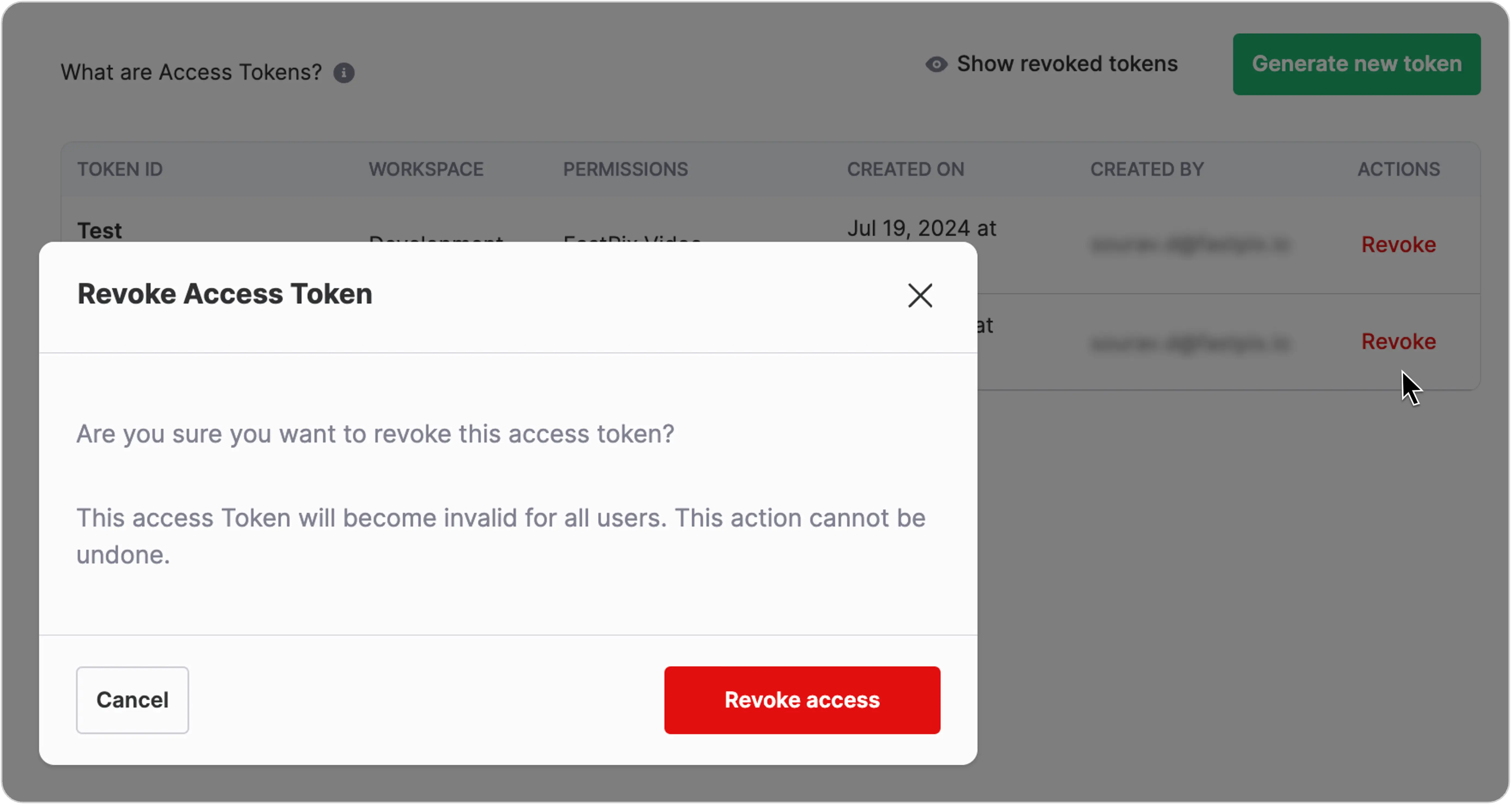
Admin privileges
Access to the access tokens settings page is restricted to administrators within the FastPix organization. Members or regular users won’t have access. Therefore, admins play a crucial role in managing tokens and ensuring their proper use.
Authenticating API requests
FastPix’s API endpoints require authentication via HTTP Basic Auth. Here’s how it works:
-
Base64 encoding: Concatenate the Access Token ID (
username
) and Secret Key (password
) using a colon (“:”). Then base64 encode the resulting string. -
Authorization header: The header value should start with “Basic” followed by a space and the base64 encoded result from previous step.
The header value follows this format - Authorization: Basic base64(username:password)
Example request with cURL
Here’s an example of authenticating a request using cURL:
curl -X POST 'https://v1.fastpix.io/on-demand'
--user {Access Token ID}:{Secret Key} \
-H 'Content-Type: application/json' \
-d '{
"inputs": [
{
"type": "video",
"url": "https://static.fastpix.io/gtv-videos-bucket/sample/ForBiggerJoyrides.mp4",
"startTime": 0,
"endTime": 60
},
{
"type": "watermark",
"url": "https://static.fastpix.io/watermark-4k.png",
"placement": {
"xAlign": "left",
"xMargin": "10%",
"yAlign": "top",
"yMargin": "10%"
}
}
],
"metadata": {
"key1": "value1"
},
"createSubtitles": {
"name": "name",
"metadata": {
"key1": "value1"
},
"languageCode": "en-us"
},
"accessPolicy": "public",
"mp4Support": "capped_4k",
"optimizeAudio": true,
"maxResolution": "1080p"
}'
PLEASE NOTE
Remember to handle the base64 encoding of the username and password in your server-side code, allowing the library to manage the header details.
Updated 3 months ago