Monitor HLS.js (Web)
Key features:
- Track viewer engagement: Gain insights into how users interact with your videos.
- Monitor playback quality: Ensure video streaming by monitoring real-time metrics, including bitrate, buffering, startup performance, render quality, and playback failure errors.
- Error management: Identify and resolve playback failures quickly with detailed error reports.
- Customizable analytics: Configure tracking attributes to meet your business requirements, providing flexibility and scalability.
- Centralized dashboard: Visualize and compare metrics on the FastPix dashboard to make data-driven decisions.
Step 1: Installation and setup
This package can be installed using npm, a CDN, or your preferred package manager.
using npm:
npm install @fastpix/data-core
Using CDN:
<script src="https://cdn.jsdelivr.net/npm/@fastpix/data-core@latest"></script>
PLEASE NOTE
Ensure that a package based on HLS Player is installed or accessible via a CDN.
Initialize FastPix Data SDK
To start tracking and analyzing video performance using FastPix, you need to initialize the FastPix Data SDK with your unique Workspace key. Follow these steps:
- Log into your FastPix Dashboard and go to the Workspaces section.
- Once you've identified the correct workspace, copy the Workspace Key associated with it. This key is essential for client-side monitoring and should be included in the JavaScript code on every webpage where you want to track video performance and analytics.
Understand what is a Workspace.
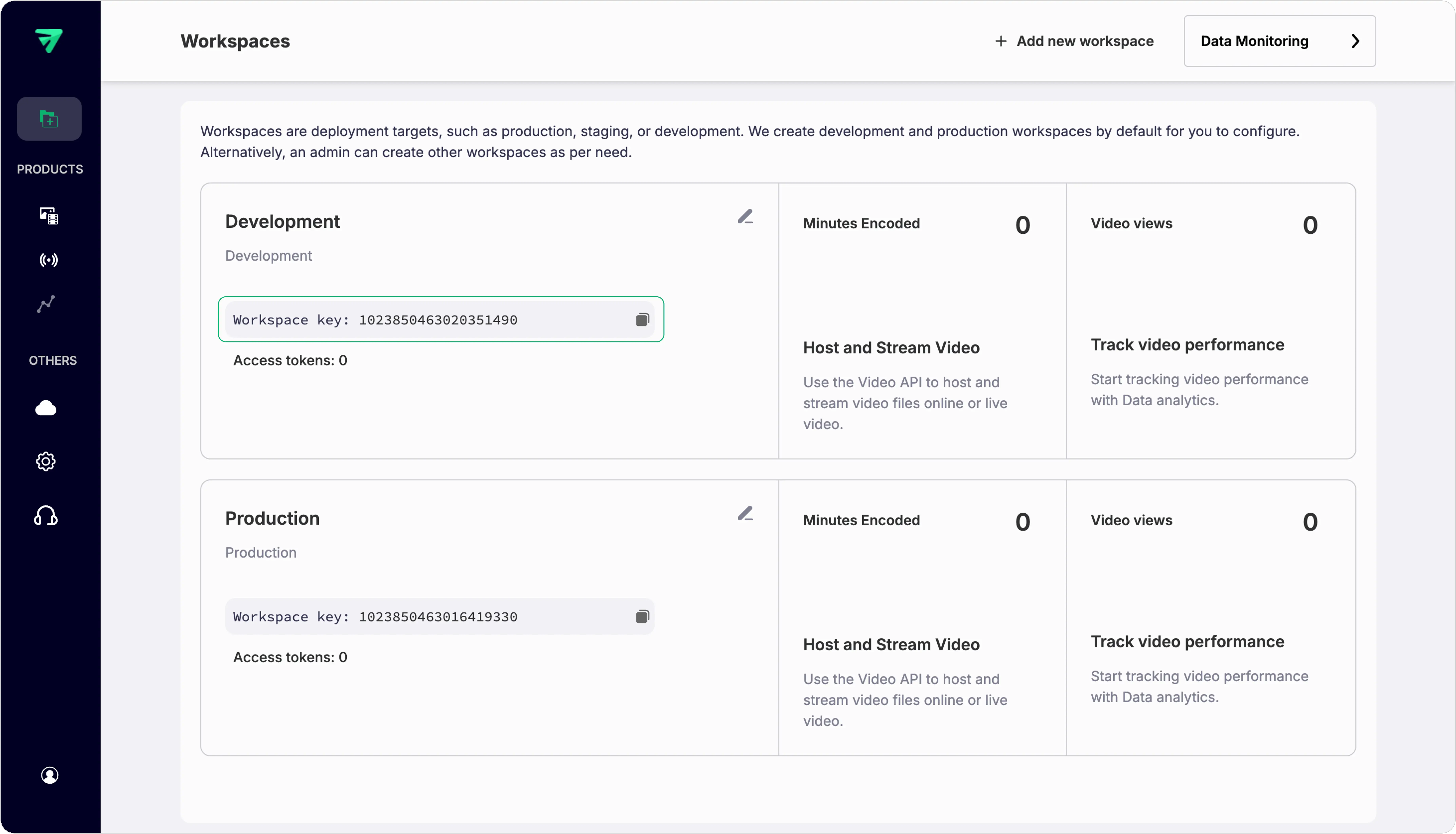
Step 2: Import the SDK
import fastpixMetrix from "@fastpix/data-core"
Step 3: Basic integration
The workspace_id
is a mandatory field that must be provided. In addition, install the hls.js package, import the Hls instance, and attach it to the HTML5 video element. Pass both the Hls instance and the Hls constructor function, along with custom metadata, to the fastpixMetrix.tracker function.
Once the player has loaded the URL and playback has started, the SDK will then begin tracking the analytics.
// Import HLS.js library for video streaming
import Hls from "hls.js";
import fastpixMetrix from "@fastpix/data-core";
// Reference to the video element
const videoPlayerElement = document.getElementById('video-player');
const initializationTime = fastpixMetrix.utilityMethods.now();
// Create a new HLS instance
const hlsPlayerInstance = new Hls();
hlsPlayerInstance.loadSource(""); // Load the video stream
hlsPlayerInstance.attachMedia(videoPlayerElement);
// Custom metadata for tracking
const trackingData = {
workspace_id: "WORKSPACE_KEY", // Mandatory field for FastPix integration, replace with your actual workspace key
player_name: "PLAYER_NAME", // A unique identifier for this player instance (e.g., "MyVideoPlayer1")
player_init_time: initializationTime, // The timestamp when the player was initialized, useful for analytics
video_title: "VIDEO_TITLE", // The title of the video being played (e.g., "My Amazing Video")
video_id: "VIDEO_ID", // Unique identifier for the video (e.g., from your CMS or database)
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Add any additional metadata
};
// Pass both `hlsPlayerInstance` and `Hls` to the FastPix tracker for correct tracking
fastpixMetrix.tracker(videoPlayerElement, {
debug: false, // Set to true to enable debug logs in the console
hlsjs: hlsPlayerInstance, // Pass the `hlsPlayerInstance` created above
Hls, // Pass the `Hls` constructor (imported)
data: trackingData, // Attach custom metadata for analytics and tracking
});
// To stop monitoring call when destroying the HLS player
// hlsPlayerInstance.fp.destroy();
PLEASE NOTE
When configuring the SDK, include both the hls instance and the Hls constructor in the options.
After successfully completing Step 3, you can track viewer metrics in the FastPix dashboard once playback ends. Steps 4, 5, and 6 are optional and can be utilized as needed to enhance your integration.
Step 4: Enhance tracking with user passable metadata
Check out the user-passable metadata documentation to see the metadata supported by FastPix. You can use custom metadata fields like custom_1 to custom_10 for your business logic, giving you the flexibility to pass any required values. Named attributes, such as video_title and video_id, can be passed directly as they are.
// Import HLS.js library for video streaming
import Hls from "hls.js";
import fastpixMetrix from "@fastpix/data-core";
// Reference to the video element
const videoPlayerElement = document.getElementById('video-player');
const initializationTime = fastpixMetrix.utilityMethods.now(); // Get the current timestamp for player initialization
// Create a new HLS instance
const hlsPlayerInstance = new Hls();
hlsPlayerInstance.loadSource(""); // Load the video stream
hlsPlayerInstance.attachMedia(videoPlayerElement);
// Custom metadata for tracking
const trackingData = {
workspace_id: "WORKSPACE_KEY", // Unique key to identify your workspace (replace with your actual workspace key)
player_name: "Main Video Player", // A custom name or identifier for this video player instance
player_init_time: initializationTime, // Timestamp of when the player was initialized (useful for tracking performance metrics)
video_title: "Test Content", // Title of the video being played (replace with the actual title of your video)
video_id: "video1234", // A unique identifier for the video (replace with your actual video ID for tracking purposes)
viewer_id: "user12345", // A unique identifier for the viewer (e.g., user ID, session ID, or any other unique value)
video_content_type: "series", // Type of content being played (e.g., series, movie, etc.)
video_stream_type: "on-demand", // Type of streaming (e.g., live, on-demand)
// Custom fields for additional business logic
custom_1: "", // Use this field to pass any additional data needed for your specific business logic
custom_2: "" // Use this field to pass any additional data needed for your specific business logic
// Add any additional metadata here if needed
};
// Pass both `hlsPlayerInstance` and `Hls` to the FastPix tracker for correct tracking
fastpixMetrix.tracker(videoPlayerElement, {
debug: false, // Set to true to enable debug logs in the console
hlsjs: hlsPlayerInstance, // Pass the `hlsPlayerInstance` create above
Hls, // Pass the `Hls` constructor (imported)
data: trackingData, // Attach custom metadata for analytics and tracking
});
DEVELOPMENT TIP
Keep metadata consistent across different video loads to make comparison easier in your analytics dashboard.
Step 5: Advanced configurations with HLS.js
Enhancing your video player with advanced options can significantly improve user experience and data tracking. Below are key configurations you can implement when using the FastPix SDK with your HLS.js player instance.
Attribute | Description | Type | Example usage |
---|---|---|---|
disableCookies | FastPix Data SDK uses cookies by default to track playback across page views and to identify unique viewers. If your application is not intended to collect cookies, you can disable this feature by setting disableCookies: true . This ensures that no cookies are set during the user's session, enhancing privacy and compliance with user preferences. | Boolean | disableCookies: true |
respectDoNotTrack | Set to true to honor users' privacy preferences regarding the 'Do Not Track' setting. | Boolean | respectDoNotTrack: true |
automaticErrorTracking | FastPix automatically tracks errors that occur during playback failures. To disable this feature, set automaticErrorTracking to false. This allows you to have more control over which errors are considered fatal and helps you manage error reporting according to your application's needs. | Boolean | automaticErrorTracking: false |
debug | Set to true to enable debug logs in the console for troubleshooting purposes. | Boolean | debug: true |
Example:
// Reference to the video element
const videoPlayerElement = document.getElementById("video-player");
// Configuration for FastPix tracker
const trackingData = {
debug: true, // Set to true to enable debug logs in the console
hlsjs: hls, // Pass the HLS.js instance
Hls: Hls, // Pass the Hls constructor (imported)
disableCookies: true, // Set to true to disable cookies for tracking sessions and unique viewers
respectDoNotTrack: true, // Set to true to honor users' 'Do Not Track' preferences
automaticErrorTracking: false, // Set to false to disable automatic tracking of fatal errors
data: {
workspace_id: "WORKSPACE_KEY", // Replace with your actual workspace key
// ... add other metadata as needed
},
};
// Initialize the FastPix tracker with the configuration
fastpixMetrix.tracker(videoPlayerElement, trackingData);
Step 6: Emit custom events
Advanced error reporting and contextual tracking
By default, FastPix tracks errors that occur during playback failures. However, you can also emit a custom error event for non-severe issues that arise outside of these failures, allowing you to provide additional context for tracking purposes.
// videoPlayerElement is the HTML5 <video> element representing your video player.
const videoPlayerElement = document.getElementById("video-player");
videoPlayerElement.fp.dispatch("error", {
player_error_code: 1024, // Custom error code
player_error_message: "Description of error", // Generalized error message
player_error_context: "Additional context for the error", // Instance-specific information
});
TIP
Use custom error codes and messages that are meaningful for your debugging process to streamline troubleshooting.
Changing video streams in player
Effective video view tracking is crucial to track multiple videos back-to-back in the same player in your application. You can reset tracking when loading a new source, such as in video series or episodic content or in cases where the user wants to play any other video.
Emitting avideoChange
event:
To inform the FastPix SDK of a new view, emit a videoChange event immediately after loading the new video source. Include relevant metadata about the new video:
// videoPlayerElement is the HTML5 <video> element representing your video player.
const videoPlayerElement = document.getElementById("video-player");
videoPlayerElement.fp.dispatch("videoChange", {
video_id: "abc345", // Unique identifier for the new video
video_title: "My Other Great Video", // Title of the new video
video_series: "Weekly Great Videos", // Series name if applicable
// Additional metadata can be included here
});
PLEASE NOTE
Always ensure that this event is dispatched right after the new source is loaded to maintain accurate tracking.
Example to configure HLS with FastPix Data SDK
Here are platform-specific examples to help you integrate the FastPix Data SDK with your HLS player. Use the following React or JavaScript or HTML code into your application:
import React, { useEffect, useRef } from "react";
import Hls from "hls.js"; // Import HLS.js library for video streaming
import fastpixMetrix from "@fastpix/data-core";
export default function VideoPlayer() {
const videoElementRef = useRef(null);
// Replace with your actual stream URL
const videoSourceUrl = "https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8"; // Your HLS stream URL
useEffect(() => {
let hlsInstance;
// Check if the videoElementRef.current is available before proceeding
if (videoElementRef.current) {
const videoElement = videoElementRef.current;
const playerInitTime = fastpixMetrix.utilityMethods.now(); // Get player initialization time
// Check if the video element can play HLS natively (Safari support)
if (videoElement.canPlayType("application/vnd.apple.mpegurl")) {
// For Safari, which has native HLS support
videoElement.src = videoSourceUrl;
} else if (Hls.isSupported()) {
// For other browsers, initialize Hls.js
hlsInstance = new Hls();
hlsInstance.loadSource(videoSourceUrl); // Load the video stream
hlsInstance.attachMedia(videoElement); // Attach the stream to the video element
// Custom metadata to be passed for tracking
const customData = {
workspace_id: "WORKSPACE_KEY", // Mandatory field for FastPix integration, replace with your actual workspace key
player_name: "PLAYER_NAME", // A unique identifier for this player instance (e.g., "MyVideoPlayer1")
player_init_time: playerInitTime, // The timestamp when the player was initialized, useful for analytics
video_title: "VIDEO_TITLE", // The title of the video being played (e.g., "My Amazing Video")
video_id: "VIDEO_ID", // Unique identifier for the video (e.g., from your CMS or database)
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Add any additional metadata here
};
// pass both `hlsInstance` and `Hls` to the FastPix tracker for correct tracking
fastpixMetrix.tracker(videoElement, {
debug: false,
hlsjs: hlsInstance, // Pass the `hlsInstance` created above
Hls, // Pass the `Hls` constructor (imported)
data: customData,
});
}
}
// Cleanup on component unmount
return () => {
if (hlsInstance) {
hlsInstance.destroy(); // Clean up HLS.js instance when the component is unmounted
}
};
}, [videoElementRef]);
return(
<video controls ref={videoElementRef} style={{ width: "100%", maxWidth: "500px" }} />
);
}
import Hls from "hls.js";
import fastpixMetrix from "@fastpix/data-core";
const videoElement = document.getElementById('video-player'); // Video element reference
const videoSourceUrl = "https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8"; // Your HLS stream URL
// Ensure the video element is available
if (videoElement) {
let hlsInstance;
const playerInitTime = fastpixMetrix.utilityMethods.now(); // Get player initialization time
// Check if the video element can play HLS natively (Safari support)
if (videoElement.canPlayType("application/vnd.apple.mpegurl")) {
// For Safari, which has native HLS support
videoElement.src = videoSourceUrl;
} else if (Hls.isSupported()) {
// For other browsers, initialize Hls.js
hlsInstance = new Hls();
hlsInstance.loadSource(videoSourceUrl); // Load the video stream
hlsInstance.attachMedia(videoElement); // Attach the stream to the video element
// Custom metadata to be passed for tracking
const customData = {
workspace_id: "WORKSPACE_KEY", // Mandatory field for FastPix integration, replace with your actual workspace key
player_name: "PLAYER_NAME", // A unique identifier for this player instance (e.g., "MyVideoPlayer1")
player_init_time: playerInitTime, // The timestamp when the player was initialized, useful for analytics
video_title: "VIDEO_TITLE", // The title of the video being played (e.g., "My Amazing Video")
video_id: "VIDEO_ID", // Unique identifier for the video (e.g., from your CMS or database)
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Add any additional metadata here
};
// pass both `hlsInstance` and `Hls` to the FastPix tracker for correct tracking
fastpixMetrix.tracker(videoElement, {
debug: false,
hlsjs: hlsInstance, // Pass the `hlsInstance` created above
Hls, // Pass the `Hls` constructor (imported)
data: customData,
});
}
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="https://cdn.jsdelivr.net/npm/@fastpix/data-core@latest"></script>
<script src="https://cdn.jsdelivr.net/npm/hls.js@latest"></script> <!-- Use the HLS CDN as preferred -->
<title>FastPix Hls Analytics</title>
</head>
<body>
<video id="video-player" autoplay controls width="660" height="380"></video>
<script>
// Get the video element reference
const videoElement = document.getElementById('video-player'); // Video element reference
const videoSourceUrl = "https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8"; // Your HLS stream URL
// Ensure the video element is available
if (videoElement) {
let hlsInstance;
const playerInitTime = fastpixMetrix.utilityMethods.now(); // Get player initialization time
// Check if the video element can play HLS natively (Safari support)
if (videoElement.canPlayType("application/vnd.apple.mpegurl")) {
videoElement.src = videoSourceUrl;
} else if (Hls.isSupported()) {
// For other browsers, initialize Hls.js
hlsInstance = new Hls();
hlsInstance.loadSource(videoSourceUrl); // Load the video stream
hlsInstance.attachMedia(videoElement); // Attach the stream to the video element
if (window && window.fastpixMetrix) {
const initFastPixHlsAnalytics = window.fastpixMetrix
// Custom metadata to be passed for tracking
const customData = {
workspace_id: "WORKSPACE_KEY", // Mandatory field for FastPix integration, replace with your actual workspace key
player_name: "PLAYER_NAME", // A unique identifier for this player instance (e.g., "MyVideoPlayer1")
player_init_time: playerInitTime, // The timestamp when the player was initialized, useful for analytics
video_title: "VIDEO_TITLE", // The title of the video being played (e.g., "My Amazing Video")
video_id: "VIDEO_ID", // Unique identifier for the video (e.g., from your CMS or database)
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Add any additional metadata
};
// Pass both `hlsInstance` and `Hls` to the FastPix tracker for correct tracking
initFastPixHlsAnalytics.tracker(videoElement, {
debug: false,
hlsjs: hlsInstance, // Pass the `hlsInstance` created above
Hls, // Pass the `Hls` constructor (imported)
data: customData,
});
}
}
}
</script>
</body>
</html>
Updated 3 days ago