Monitor Shaka player (Web)
The FastPix Data SDK with Shaka player enables tracking of player analytics, including key video performance metrics such as user interactions, playback quality, and performance.
Key features:
- Track viewer engagement: Gain insights into how users interact with your videos.
- Monitor playback quality: Ensure video streaming by monitoring real-time metrics, including bitrate, buffering, startup performance, render quality, and playback failure errors.
- Error management: Identify and resolve playback failures quickly with detailed error reports.
- Customizable analytics: configure tracking attributes to meet your business requirements, providing flexibility and scalability.
- Centralized dashboard: Visualize and compare metrics on the FastPix dashboard to make data-driven decisions.
Step 1
Installation and setup
Step 2
Import the SDK
Step 3
Basic integration
Step 4
Enhance tracking with metadata
Step 5
Advanced configurations
Step 6
Emit custom events
Prerequisites
To track and analyze video performance, initialize the FastPix Data SDK with your Workspace key.
-
Log into your FastPix Dashboard and go to the Workspaces section.
-
Once you've identified the correct workspace, copy the Workspace Key associated with it. This key is essential for client-side monitoring and should be included in the JavaScript code on every webpage where you want to track video performance and analytics.
Understand what is a Workspace.
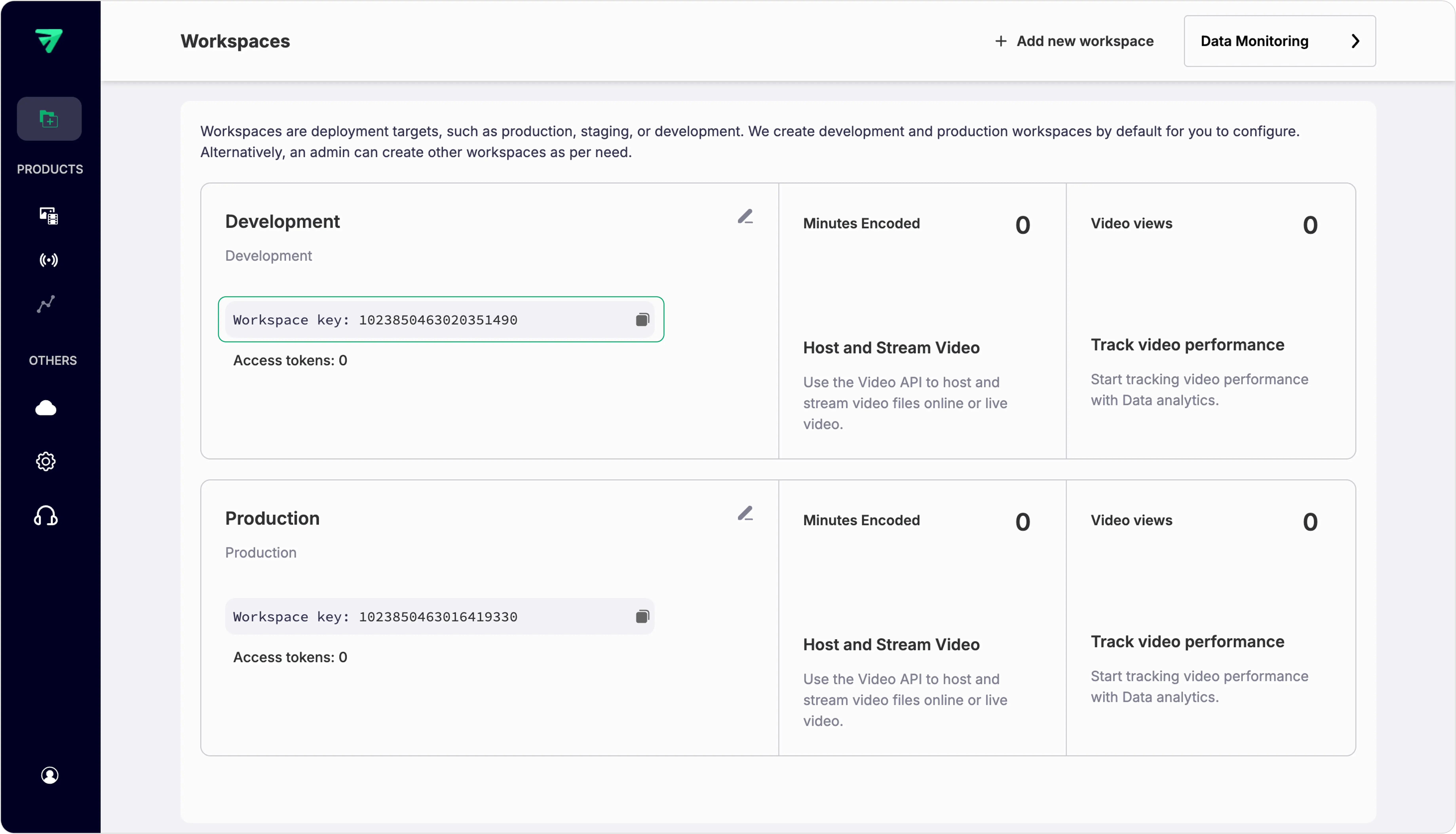
Step 1: Installation and setup
This package can be installed using npm, a CDN, or your preferred package manager:
Using npm:
npm install "@fastpix/video-data-shakaplayer"
Using CDN:
<script src="https://cdn.jsdelivr.net/npm/@fastpix/video-data-shakaplayer@latest"></script>
PLEASE NOTE
Ensure that a package based on Shaka Player is installed or accessible via a CDN.
Step 2: Import the SDK
import loadShakaPlayer from "@fastpix/video-data-shakaplayer";
Step 3: Basic integration
Ensure that the workspace_id
is provided, as it is a mandatory field for FastPix integration, uniquely identifying your workspace. Install and import Shaka Player into your project, and create an HTML5 <video>
element to bind it to.
Once the video element is set up, initialize the Shaka Player instance and associate it with the video element. Use the loadShakaPlayer
function to pass the Shaka Player instance, player metadata, and the shaka instance, enabling FastPix to track playback data.
Once the video URL is loaded and playback has started, the SDK will begin tracking the analytics.
import loadShakaPlayer from "@fastpix/video-data-shakaplayer";
import shaka from "shaka-player"; // Import Shaka Player
// Initialize player setup
const initTime = loadShakaPlayer.utilityMethods.now(); // Captures the exact timestamp of player initialization
const videoElement = document.getElementById("video-player"); // Select the HTML5 video element for Shaka Player
const player = new shaka.Player(videoElement); // Create a Shaka Player instance bound to the video element
// Define player metadata
const playerMetadata = {
workspace_id: "WORKSPACE_KEY", // Mandatory field for FastPix integration, replace with your actual workspace key
player_name: "PLAYER_NAME", // A unique identifier for this player instance (e.g., "MyVideoPlayer1")
player_init_time: initTime, // The timestamp when the player was initialized, useful for analytics
video_title: "VIDEO_TITLE", // The title of the video being played (e.g., "My Amazing Video")
video_id: "VIDEO_ID", // Unique identifier for the video (e.g., from your CMS or database)
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Additional metadata
};
// Configure FastPix data integration
const fastPixShakaIntegration = loadShakaPlayer(
player, // The Shaka Player instance managing playback
{
debug: false, // Optional flag; set to true to enable debug logs for troubleshooting
data: playerMetadata,
},
shaka // Pass the imported Shaka Player instance for proper integration
);
// Load the video content
const videoUrl = "https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8"; // Replace with your video manifest URL
player
.load(videoUrl) // Load the video manifest URL into the Shaka Player
.then(() => {
// Successfully loaded the manifest; FastPix will now begin tracking playback data
console.log("Video manifest loaded successfully.");
})
.catch((error) => {
// Handle errors that occur while loading the video manifest
fastPixShakaIntegration.handleLoadError(error); // Notify FastPix of the error
console.error("Error loading video manifest:", error); // Log the error for debugging
});
// Use these methods to destroy fastpix data sdk and shakaplayer:
// player.destroy() - Destroys the Shaka Player
// player.fp.destroy() - Ends FastPix tracking
PLEASE NOTE
To end FastPix data tracking, always call
player.fp.destroy()
when callingplayer.destroy()
(Shaka Player instance). This ensures proper cleanup of both Shaka Player and FastPix data tracking.
// player is the instance returned by `new shaka.Player`
player.fp.destroy(); // Ends FastPix tracking
player.destroy(); // Destroys the Shaka Player instance
After successfully completing Step 3, you can track viewer metrics in the FastPix dashboard once playback ends. Steps 4, 5, and 6 are optional and can be utilized as needed to enhance your integration.
Step 4: Enhance tracking with user passable metadata
Check out the user-passable metadata documentation to see the metadata supported by FastPix. You can use custom metadata fields like custom_1 to custom_10 for your business logic, giving you the flexibility to pass any required values. Named attributes, such as video_title and video_id, can be passed directly as they are.
import loadShakaPlayer from "@fastpix/video-data-shakaplayer";
import shaka from "shaka-player"; // Import Shaka Player
// Initialize player setup
const initTime = loadShakaPlayer.utilityMethods.now(); // Captures the exact timestamp of player initialization
const videoElement = document.getElementById("video-player"); // Select the HTML5 video element for Shaka Player
const player = new shaka.Player(videoElement); // Create a Shaka Player instance bound to the video element
// Define player metadata
const playerMetadata = {
workspace_id: "WORKSPACE_KEY", // Mandatory field for FastPix integration, replace with your actual workspace key
player_name: "PLAYER_NAME", // A unique identifier for this player instance (e.g., "MyVideoPlayer1")
player_init_time: initTime, // The timestamp when the player was initialized, useful for analytics
video_title: "Test Content", // Title of the video being played (replace with the actual title of your video)
video_id: "f01a98s76t90p88i67x", // A unique identifier for the video (replace with your actual video ID for tracking purposes)
viewer_id: "user12345", // A unique identifier for the viewer (e.g., user ID, session ID, or any other unique value)
video_content_type: "series", // Type of content being played (e.g., series, movie, etc.)
video_stream_type: "on-demand", // Type of streaming (e.g., live, on-demand)
// Custom fields for additional business logic
custom_1: "", // Use this field to pass any additional data needed for your specific business logic
custom_2: "", // Use this field to pass any additional data needed for your specific business logic
// Additional metadata
};
// Configure FastPix data integration
const fastPixShakaIntegration = loadShakaPlayer(
player, // The Shaka Player instance managing playback
{
debug: false, // Optional flag; set to true to enable debug logs for troubleshooting
data: playerMetadata,
},
shaka, // Pass the imported Shaka Player instance for proper integration
);
// Load the video content
const videoUrl =
"https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8"; // Replace with your video manifest URL
player
.load(videoUrl) // Load the video manifest URL into the Shaka Player
.then(() => {
// Successfully loaded the manifest; FastPix will now begin tracking playback data
console.log("Video manifest loaded successfully.");
})
.catch((error) => {
// Handle errors that occur while loading the video manifest
fastPixShakaIntegration.handleLoadError(error); // Notify FastPix of the error
console.error("Error loading video manifest:", error); // Log the error for debugging
});
DEVELOPMENT TIP
Keep metadata consistent across different video loads to make comparison easier in your analytics dashboard.
Step 5: Advanced configurations with FastPix Data SDK
Enhancing your Shaka Player with advanced options can significantly improve user experience and data tracking. Below are key configurations you can implement when using the FastPix SDK with your Shaka Player instance.
Attribute | Description | Type | Example Usage |
---|---|---|---|
disableCookies | FastPix Data SDK uses cookies by default to track playback across page views and to identify unique viewers. If your application is not intended to collect cookies, you can disable this feature by setting disableCookies: true . This ensures that no cookies are set during the user's session, enhancing privacy and compliance with user preferences. | Boolean | disableCookies: true |
respectDoNotTrack | Set to true to honor users' privacy preferences regarding the 'Do Not Track' setting. | Boolean | respectDoNotTrack: true |
automaticErrorTracking | FastPix automatically tracks errors that occur during playback failures. To disable this feature, set automaticErrorTracking to false. This allows you to have more control over which errors are considered fatal and helps you manage error reporting according to your application's needs. | Boolean | automaticErrorTracking: false |
debug | Set to true to enable debug logs in the console for troubleshooting purposes. | Boolean | debug: true |
Example:
// player is the instance returned by `new shaka.Player`
const fastPixShakaIntegration = loadShakaPlayer(
player, // The Shaka Player instance managing playback
{
debug: false, // Optional flag; set to true to enable debug logs for troubleshooting
disableCookies: true, // Set to true to disable cookies for tracking sessions and unique viewers
respectDoNotTrack: true, // Set to true to honor users' 'Do Not Track' preferences
automaticErrorTracking: false, // Set to false to disable automatic tracking of fatal errors
data: {
workspace_id: "WORKSPACE_KEY", // Mandatory field for FastPix integration, replace with your actual workspace key
// Additional metadata
},
},
shaka, // Pass the imported Shaka Player instance
);
Step 6: Emit custom events
Advanced error reporting and contextual tracking
By default, FastPix tracks errors that occur during playback failures. However, you can also emit a custom error event for non-severe issues that arise outside of these failures, allowing you to provide additional context for tracking purposes.
// player is the instance returned by `new shaka.Player`
player.fp.dispatch("error", {
player_error_code: 1008, // Custom error code
player_error_message: "Description of error", // Generalized error message
player_error_context: "Additional context for the error", // Instance-specific information
});
TIP
Use custom error codes and messages that are meaningful for your debugging process to streamline troubleshooting.
Changing video streams in player
Effective video view tracking is crucial to track multiple videos back-to-back in the same player in your application. You can reset tracking when loading a new source, such as in video series or episodic content or in cases where the user wants to play any other video.
Emitting avideoChange
event:
To inform the FastPix SDK of a new view, emit a videoChange event immediately after loading the new video source. Include relevant metadata about the new video:
// player is the instance returned by `new shaka.Player`
player.fp.dispatch("videoChange", {
video_id: "abc345", // Unique identifier for the new video
video_title: "My Other Great Video", // Title of the new video
video_series: "Weekly Great Videos", // Series name if applicable
// ... and other metadata
});
PLEASE NOTE
Always ensure that this event is dispatched right after the new source is loaded to maintain accurate tracking.
Example to configure Shaka Player with FastPix Data SDK
Here are platform-specific examples to help you integrate the FastPix Data SDK with your Shaka Player. Use the following React or JavaScript or HTML code into your application:
import React, { useEffect, useRef } from "react";
import loadShakaPlayer from "@fastpix/video-data-shakaplayer";
import shaka from "shaka-player"; // Import Shaka Player
const ShakaPlayer = () => {
const videoElementRef = useRef(null); // Ref to the video element
useEffect(() => {
const initTime = loadShakaPlayer.utilityMethods.now(); // Captures the player initialization time
const videoElement = videoElementRef.current; // Access video element through ref
const player = new shaka.Player(videoElement); // Shaka Player instance bound to the video element
// Define player metadata
const playerMetadata = {
workspace_id: "WORKSPACE_KEY", // Replace with your workspace key
player_name: "PLAYER_NAME", // A unique identifier for the player instance
player_init_time: initTime, // The time the player was initialized
video_title: "VIDEO_TITLE", // Title of the video being played for analytics
video_id: "VIDEO_ID", // Unique identifier for the video
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Additional metadata can be added here
};
// Configure FastPix data integration
const fastPixShakaIntegration = loadShakaPlayer(
player,
{
debug: false, // Set to true to enable debug logs
data: playerMetadata, // Pass metadata object
},
shaka, // The Shaka Player instance (mandatory field)
);
// Load the video content
const videoUrl =
"https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8"; // Replace with your video manifest URL
player
.load(videoUrl) // Load the video manifest into the Shaka Player
.then(() => {
// Successfully loaded the manifest. FastPix data will begin tracking.
})
.catch((error) => {
fastPixShakaIntegration.handleLoadError(error);
});
// Cleanup: Destroy the player instance and stop FastPix data tracking when the component unmounts
return () => {
player.fp.destroy(); // Ends FastPix tracking
player.destroy(); // Destroys the Shaka Player instance
};
}, []); // Empty dependency array ensures this runs only once when the component mounts
return (
<div>
<video
ref={videoElementRef}
id="my-player"
autoPlay
width="100%"
controls
></video>
</div>
);
};
export default ShakaPlayer;
import loadShakaPlayer from "@fastpix/video-data-shakaplayer";
import shaka from "shaka-player"; // Import Shaka Player
// Initialize player setup
const initTime = loadShakaPlayer.utilityMethods.now(); // Captures the player initialization time
const videoElement = document.getElementById("video-player"); // HTML5 video element for Shaka Player
const player = new shaka.Player(videoElement); // Shaka Player instance bound to the video element
// Define player metadata
const playerMetadata = {
workspace_id: "WORKSPACE_KEY", // Replace with your workspace key
player_name: "PLAYER_NAME", // A unique identifier for the player instance
player_init_time: initTime, // The time the player was initialized
video_title: "VIDEO_TITLE", // Title of the video being played for analytics
video_id: "VIDEO_ID", // Unique identifier for the video
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Additional metadata can be added here
};
// Configure FastPix data integration
const fastPixShakaIntegration = loadShakaPlayer(
player,
{
debug: false, // Set to true to enable debug logs
data: playerMetadata, // Pass metadata object
},
shaka, // The Shaka Player instance (mandatory field)
);
// Load the video content
const videoUrl =
"https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8"; // Replace with your video manifest URL
player
.load(videoUrl) // Load the video manifest into the Shaka Player
.then(() => {
// Successfully loaded the manifest. FastPix data will begin tracking.
})
.catch((error) => {
fastPixShakaIntegration.handleLoadError(error);
});
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="https://cdnjs.cloudflare.com/ajax/libs/shaka-player/4.9.9/shaka-player.compiled.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@fastpix/video-data-shakaplayer@latest"></script>
<title>FastPix ShakaPlayer Analytics</title>
</head>
<body>
<div>
<video id="video-player" autoplay width="100%" controls></video>
</div>
<script>
if (window && window.loadShakaPlayer) {
// Initialize player setup
const initTime = window.loadShakaPlayer.utilityMethods.now(); // Captures the player initialization time
const videoElement = document.getElementById("video-player"); // HTML5 video element for Shaka Player
const player = new shaka.Player(videoElement); // Shaka Player instance bound to the video element
// Define player metadata
const playerMetadata = {
workspace_id: "WORKSPACE_KEY", // Replace with your workspace key
player_name: "PLAYER_NAME", // A unique identifier for the player instance
player_init_time: initTime, // The time the player was initialized
video_title: "VIDEO_TITLE", // Title of the video being played for analytics
video_id: "VIDEO_ID", // Unique identifier for the video
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Additional metadata can be added here
};
// Configure FastPix data integration
const fastPixShakaIntegration = window.loadShakaPlayer(
player, {
debug: false, // Set to true to enable debug logs
data: playerMetadata, // Pass metadata object
},
shaka // The Shaka Player instance (mandatory field)
);
// Load the video content
const videoUrl = "https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8"; // Replace with your video manifest URL
player
.load(videoUrl) // Load the video manifest into the Shaka Player
.then(() => {
// Successfully loaded the manifest. FastPix data will begin tracking.
})
.catch((error) => {
fastPixShakaIntegration.handleLoadError(error);
});
}
</script>
</body>
</html>
Changelog
All notable changes to the Shaka Player Video Data SDK is documented below.
Current version
v1.0.3
Fixed some of the minor issues related to Shaka Player and video analytics.
Previous versions
v1.0.2
- Upgraded the Video Data Core SDK to the latest version.
- Updated readme.md with a redirection link for supported dimension parameters.
v1.0.1
Updated Video Data Core SDK with minor fixes and improvements.
v1.0.0
Added:
- Enabled video performance tracking using the FastPix Data SDK for Shaka Player, providing detailed insights into user engagement, playback quality, and real-time streaming diagnostics.
- Included error management and reporting capabilities for tracking playback issues specific to Shaka Player.
- Supports customizable behavior, such as disabling cookies, respecting
Do Not Track
settings, and configuring advanced error handling for Shaka Player-specific events. - Added custom metadata support to allow users to pass optional fields (
video_id
,video_title
,video_duration
, etc.) for enhanced tracking and reporting. - Introduced event tracking for
videoChange
to handle seamless metadata updates during playback transitions within Shaka Player.
Updated 7 days ago