Monitor Video.js (Web)
The FastPix Data SDK for Video.js monitors and analyzes key Video.js player metrics, providing actionable insights to optimize video streaming performance and deliver a smoother viewing experience.
Key features:
- See how users interact with your videos.
- Track real-time metrics like bitrate, buffering, startup, render quality, and failures.
- Get detailed error reports to quickly identify and fix playback issues.
- Customize tracking attributes to suit your business needs.
- View and compare metrics in one place to support data-driven decisions.
Step 1
Installation and setup
Step 2
Import the SDK
Step 3
Basic integration
Step 4
Enhance your tracking
Step 5
Advanced configuration
Step 6
Emit custom events
Prerequisites
To track and analyze video performance, initialize the FastPix Data SDK with your Workspace key.
-
Log into your FastPix dashboard and go to the Workspaces section.
-
Copy the Workspace Key for client-side monitoring. Include this key in your JavaScript code on every page where you want to track video performance.
Understand what is a Workspace.
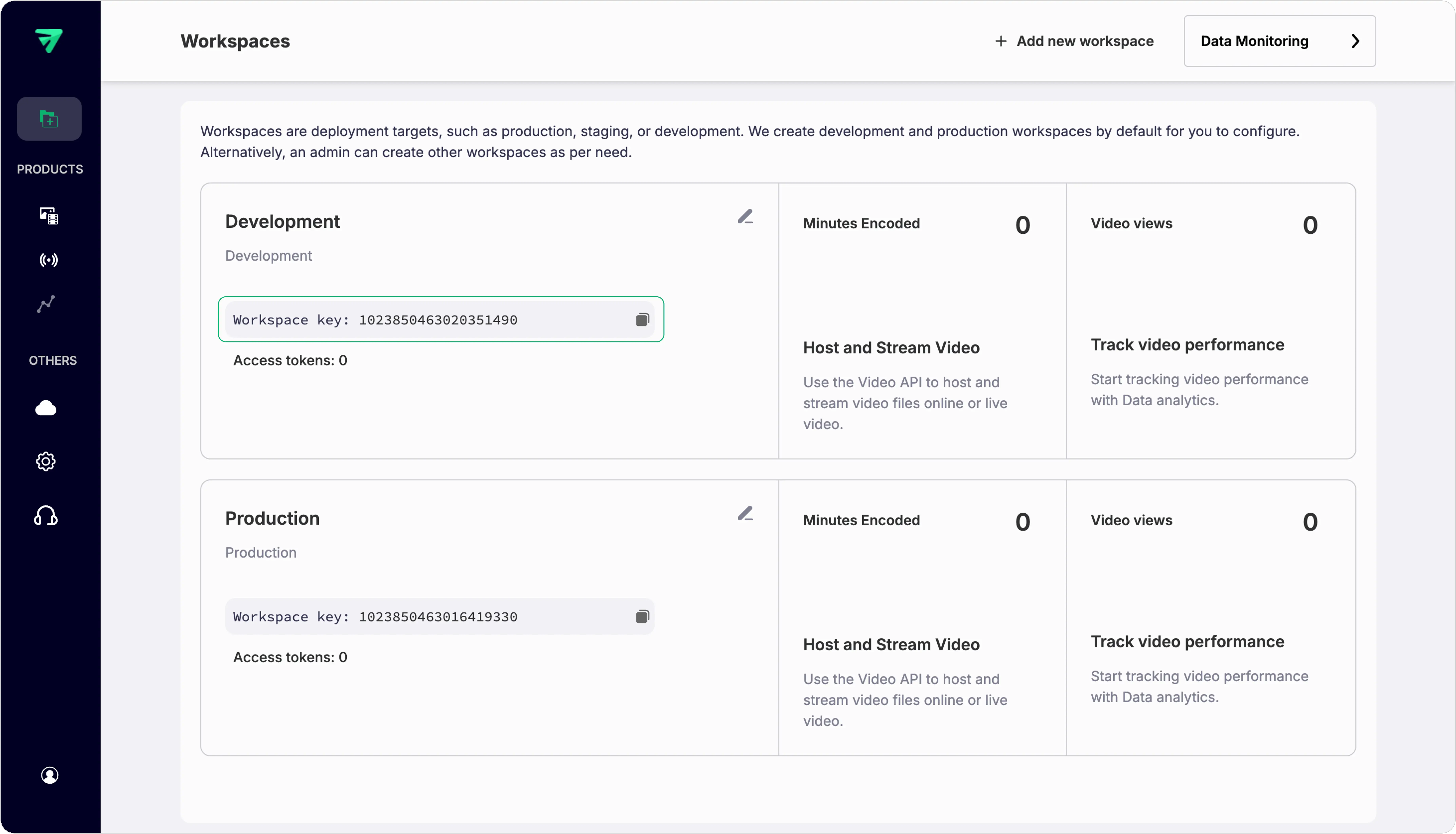
Step 1: Installation and setup
This package can be installed using npm, a CDN, or your preferred package manager.
View GitHub repoUsing npm:
npm install "@fastpix/videojs-monitor"
Using CDN:
<script src="https://cdn.jsdelivr.net/npm/@fastpix/videojs-monitor@latest"></script>
NOTE
Ensure that a package based on Video.js Player is installed or accessible via a CDN.
Step 2: Import the SDK
import initVideoJsTracking from "@fastpix/videojs-monitor"
Step 3: Basic integration
The workspace_id is a required field and must be included. Start by installing the video.js package and linking it to your HTML5 video element. Then, pass the videojs function (imported from the video.js library) along with your custom metadata to the initVideoJsTracking function.
Once the player has loaded the URL and playback has started, the SDK will then begin tracking the analytics.
// Import the Video.js library for video streaming
import videojs from "video.js";
import initVideoJsTracking from "@fastpix/videojs-monitor";
// Reference to the video element
const videoPlayerElement = document.getElementById("video-player");
// Record the player initialization time
const initializationTime = initVideoJsTracking.utilityMethods.now();
// Create a new Video.js instance for the player
const videojsInstance = videojs(videoPlayerElement);
// Custom metadata for tracking purposes
const trackingData = {
workspace_id: "WORKSPACE_KEY", // Unique key to identify your workspace (replace with your actual workspace key)
player_name: "PLAYER_NAME", // A custom name or identifier for this video player instance
player_init_time: initializationTime, // Timestamp of when the player was initialized (useful for performance tracking)
video_title: "VIDEO_TITLE", // Title of the video being played for analytics
video_id: "VIDEO_ID", // Unique identifier for the video
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Add any additional metadata if needed
};
// Initialize video.js tracking with custom configuration
initVideoJsTracking(videojsInstance, {
debug: false, // Set to true to enable debug logs in the console
videojs: videojs, // Pass the imported videojs function
data: trackingData, // Attach custom metadata for analytics and tracking
});
// Call this method to stop the monitoring.
// videojsInstance.fp.destroy();
After successfully completing Step 3, you can track viewer metrics in the FastPix dashboard once playback ends. Steps 4, 5, and 6 are optional and can be utilized as needed to enhance your integration.
Step 4: Enhance your tracking with user passable metadata
Check out the user-passable metadata documentation to see the metadata supported by FastPix. You can use custom metadata fields like custom_1 to custom_10 for your business logic, giving you the flexibility to pass any required values. Named attributes, such as video_title and video_id, can be passed directly as they are.
// Import the Video.js library for video streaming
import videojs from "video.js";
import initVideoJsTracking from "@fastpix/videojs-monitor";
// Reference to the video element
const videoPlayerElement = document.getElementById("video-player");
// Record the player initialization time
const initializationTime = initVideoJsTracking.utilityMethods.now();
// Create a new Video.js instance for the player
const videojsInstance = videojs(videoPlayerElement);
// Custom metadata for tracking
const trackingData = {
workspace_id: "WORKSPACE_KEY", // Unique key to identify your workspace (replace with your actual workspace key)
player_name: "PLAYER_NAME", // A custom name or identifier for this video player instance
player_init_time: initializationTime, // Timestamp of when the player was initialized (useful for tracking performance metrics)
video_title: "VIDEO_TITLE", // Title of the video being played (replace with the actual title of your video)
video_id: "VIDEO_ID", // A unique identifier for the video (replace with your actual video ID for tracking purposes)
viewer_id: "user12345", // A unique identifier for the viewer (e.g., user ID, session ID, or any other unique value)
video_content_type: "series", // Type of content being played (e.g., series, movie, etc.)
video_stream_type: "on-demand", // Type of streaming (e.g., live, on-demand)
// Custom fields for additional business logic
custom_1: "", // Use this field to pass any additional data needed for your specific business logic
custom_2: "", // Use this field to pass any additional data needed for your specific business logic
// Add any additional metadata
};
// Initialize video.js tracking with custom configuration
initVideoJsTracking(videojsInstance, {
debug: false, // Set to true to enable debug logs in the console
videojs: videojs, // Pass the imported videojs function
data: trackingData, // Attach custom metadata for analytics and tracking
});
// Call this method to stop the monitoring.
// videojsInstance.fp.destroy();
DEVELOPMENT TIP
Keep metadata consistent across different video loads to make comparison easier in your analytics dashboard.
Step 5: Advanced customization with FastPix Data SDK
Enhancing your video player with advanced options can significantly improve user experience and data tracking. Below are key configurations you can implement when using the FastPix SDK with your HLS.js player instance.
Attribute | Description | Type | Example Usage |
---|---|---|---|
disableCookies | FastPix Data SDK uses cookies by default to track playback across page views and to identify unique viewers. If your application is not intended to collect cookies, set disableCookies: true . This ensures no cookies are set during the user's session, enhancing privacy and compliance with user preferences. | Boolean | disableCookies: true |
respectDoNotTrack | Set to true to honor users' privacy preferences regarding the 'Do Not Track' setting. | Boolean | respectDoNotTrack: true |
automaticErrorTracking | FastPix automatically tracks errors that occur during playback failures. To disable this feature, set automaticErrorTracking to false. This gives you control over which errors are considered fatal. | Boolean | automaticErrorTracking: false |
debug | Set to true to enable debug logs in the console for troubleshooting purposes. | Boolean | debug: true |
Example:
// Import the Video.js library for video streaming
import videojs from "video.js";
import initVideoJsTracking from "@fastpix/videojs-monitor";
// Reference to the video element
const videoPlayerElement = document.getElementById("video-player");
// Create a new Video.js instance for the player
const videojsInstance = videojs(videoPlayerElement);
const trackingData = {
debug: true, // Set to true to enable debug logs in the console
videojs: videojs,
disableCookies: true, // Set to true to disable cookies for tracking sessions and unique viewers
respectDoNotTrack: true, // Set to true to honor users' 'Do Not Track' preferences
automaticErrorTracking: false, // Set to false to disable automatic tracking of fatal errors
data: {
workspace_id: "WORKSPACE_KEY", // Replace with your actual workspace key
// ... add other metadata as needed
},
};
// Initialize video.js tracking with custom configuration
initVideoJsTracking(videojsInstance, trackingData);
Step 6: Emit custom events
Advanced error reporting and contextual tracking
By default, FastPix tracks errors that occur during playback failures. However, you can also emit a custom error event for non-severe issues that arise outside of these failures, allowing you to provide additional context for tracking purposes.
// Import the Video.js library for video streaming
import videojs from "video.js";
import initVideoJsTracking from "@fastpix/videojs-monitor";
// Reference to the video element
const videoPlayerElement = document.getElementById("video-player");
// Create a new Video.js instance for the player
const videojsInstance = videojs(videoPlayerElement);
videojsInstance.fp.dispatch("error", {
player_error_code: 1024, // Custom error code
player_error_message: "Description of error", // Generalized error message
player_error_context: "Additional context for the error", // Instance-specific information
});
TIP
Use custom error codes and messages that are meaningful for your debugging process to streamline troubleshooting.
Changing video streams
When playing multiple videos back-to-back, it's important to notify the FastPix SDK whenever a new video starts to ensure accurate tracking. You should signal a new source in the following scenarios:
- The player advances to the next video in a playlist.
- The user selects a different video to play.
To inform the FastPix SDK of a new view, emit a videoChange
event immediately after loading the new video source:
// Import the Video.js library for video streaming
import videojs from "video.js";
import initVideoJsTracking from "@fastpix/videojs-monitor";
// Reference to the video element
const videoPlayerElement = document.getElementById("video-player");
// Create a new Video.js instance for the player
const videojsInstance = videojs(videoPlayerElement);
videojsInstance.fp.dispatch("videoChange", {
video_id: "abc345", // Unique identifier for the new video
video_title: "My Other Great Video", // Title of the new video
video_series: "Weekly Great Videos", // Series name if applicable
// Additional metadata can be included here
});
PLEASE NOTE
Always ensure that this event is dispatched right after the new source is loaded to maintain accurate tracking.
Example to configure Video.js with FastPix Data SDK
Here are platform-specific examples to help you integrate the FastPix Data SDK with your Video.js player. Use the following React or JavaScript or HTML code into your application:
import React from "react";
import videojs from "video.js";
import initVideoJsTracking from "@fastpix/videojs-monitor";
import "video.js/dist/video-js.css";
// Initializes a Video.js player with analytics tracking from @fastpix/videojs-monitor
export const VideoJS = () => {
// Refs for DOM element and Video.js player instance
const videoRef = React.useRef(null);
const playerRef = React.useRef(null);
// Video.js configuration options
const videoJsOptions = {
autoplay: true,
muted: true,
controls: true,
responsive: true,
fluid: true,
sources: [
{
src: "https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8",
type: "application/x-mpegURL",
},
],
};
// Initialize Video.js player on mount
React.useEffect(() => {
// Prevent re-initialization
if (!playerRef.current && videoRef.current) {
// Create <video-js> element and append it inside the wrapper
const videoElement = document.createElement("video-js");
videoElement.classList.add("vjs-big-play-centered");
videoRef.current.appendChild(videoElement);
// Initialize Video.js player
const videoPlayer = (playerRef.current = videojs(
videoElement,
videoJsOptions,
));
// Initialize FastPix Video.js monitoring plugin
initVideoJsTracking(videoPlayer, {
videojs: videojs,
data: {
workspace_id: "WORKSPACE_KEY", // Mandatory field for FastPix integration, replace with your actual workspace key
video_title: "VIDEO_TITLE", // The title of the video being played (e.g., "My Amazing Video")
player_name: "PLAYER_NAME", // A unique identifier for this player instance (e.g., "MyVideoPlayer1")
player_init_time: initVideoJsTracking?.utilityMethods.now(), // The timestamp when the player was initialized, useful for analytics
video_id: "VIDEO_ID", // Unique identifier for the video (e.g., from your CMS or database)
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Additional metadata
},
});
} else if (playerRef.current) {
playerRef.current.src(videoJsOptions.sources); // If already initialized, update the source
}
}, [videoJsOptions]);
// Cleanup on unmount
React.useEffect(() => {
return () => {
const player = playerRef.current;
if (player && !player.isDisposed()) {
player.dispose();
playerRef.current = null;
}
};
}, []);
return (
<div data-vjs-player>
{/* This is where the Video.js player will be mounted */}
<div ref={videoRef} />
</div>
);
};
export default VideoJS;
// Import the Video.js library for video streaming
import videojs from "video.js";
import initVideoJsTracking from "@fastpix/videojs-monitor";
// Reference to the video element
const videoPlayerElement = document.getElementById("video-player");
// Record the player initialization time
const initializationTime = initVideoJsTracking.utilityMethods.now();
// Create a new Video.js instance for the player
const videojsInstance = videojs(videoPlayerElement);
// Custom metadata for tracking
const trackingData = {
workspace_id: "WORKSPACE_KEY", // Unique key to identify your workspace (replace with your actual workspace key)
player_name: "PLAYER_NAME", // A custom name or identifier for this video player instance
player_init_time: initializationTime, // Timestamp of when the player was initialized (useful for tracking performance metrics)
video_title: "VIDEO_TITLE", // Title of the video being played (replace with the actual title of your video)
video_id: "VIDEO_ID", // A unique identifier for the video (replace with your actual video ID for tracking purposes)
viewer_id: "user12345", // A unique identifier for the viewer (e.g., user ID, session ID, or any other unique value)
video_content_type: "series", // Type of content being played (e.g., series, movie, etc.)
video_stream_type: "on-demand", // Type of streaming (e.g., live, on-demand)
// Custom fields for additional business logic
custom_1: "", // Use this field to pass any additional data needed for your specific business logic
custom_2: "", // Use this field to pass any additional data needed for your specific business logic
// Add any additional metadata
};
// Initialize video.js tracking with custom configuration
initVideoJsTracking(videojsInstance, {
debug: false, // Set to true to enable debug logs in the console
videojs: videojs, // Pass the imported videojs function
data: trackingData, // Attach custom metadata for analytics and tracking
});
// Call this method to stop the monitoring.
// videojsInstance.fp.destroy();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>FastPix Video Js Analytics</title>
<script src="https://vjs.zencdn.net/8.16.1/video.min.js"></script>
<!-- FastPix Video.js Monitoring Plugin -->
<script src="https://cdn.jsdelivr.net/npm/@fastpix/videojs-monitor@latest"></script>
<link href="https://vjs.zencdn.net/8.16.1/video-js.css" rel="stylesheet" />
</head>
<body>
<video id="my-player" height="300" width="800" class="video-js vjs-default-skin" autoplay controls>
<source src="https://stream.fastpix.io/027a90e4-f5e2-433d-81e5-b99ee864c3f6.m3u8" type="application/x-mpegURL" />
</video>
<script>
// Initialize the Video.js player
const player = videojs("my-player", {
autoplay: true,
controls: true,
responsive: true,
fluid: true,
preload: "auto",
});
// Initialize FastPix tracking plugin if available
if (window && window.initVideoJsTracking) {
window.initVideoJsTracking(player, {
videojs: videojs,
data: {
workspace_id: "WORKSPACE_KEY", // Mandatory field for FastPix integration, replace with your actual workspace key
video_title: "VIDEO_TITLE", // The title of the video being played (e.g., "My Amazing Video")
player_name: "PLAYER_NAME", // A unique identifier for this player instance (e.g., "MyVideoPlayer1")
player_init_time: initVideoJsTracking?.utilityMethods.now(), // The timestamp when the player was initialized, useful for analytics
video_id: "VIDEO_ID", // Unique identifier for the video (e.g., from your CMS or database)
viewer_id: "VIEWER_ID", // Unique identifier for the viewer
// Additional metadata
},
});
}
</script>
</body>
</html>
Changelog
All notable changes to the Video.js Player Video Data SDK is documented below.
Current version
v1.0.1
- Bug fix to prevent calls to getPlayerState and getPlayheadTimeInMs after the player has been destroyed.
Previous version
v1.0.0
Added:
- Integrated FastPix Data SDK with Video.js for performance tracking.
- Enables detailed insights into user engagement, playback quality, and real-time streaming diagnostics.
- Implemented robust error detection and reporting for Video.js-specific playback issues.
- Supports customizable behavior, such as disabling cookies, respecting
Do Not Track
settings, and configuring advanced error handling. - Added custom metadata support to allow users to pass optional fields (
video_id
,video_title
,video_duration
, etc.) for enhanced tracking and reporting. - Introduced event tracking for
videoChange
to handle metadata updates during playback transitions within Video.js.
Updated 3 days ago