Secured playback with JWTs
JSON Web Tokens (JWTs) are a standardized method for securely transmitting authentication and authorization data between systems. FastPix APIs use JWTs to authenticate requests, ensuring secure access to resources. Below is a comprehensive guide to creating and validating JWTs for your integration.
Use our JWT Signer: https://jwt.fastpix.app/
What is a JWT?
JSON Web Token (JWT) is an open standard (RFC 7519) for securely transmitting information between parties as a JSON object. It is compact, self-contained, and cryptographically signed, making it ideal for authentication and authorization workflows.
Structure of a JWT
A JWT consists of three components:
- Header: Defines the algorithm (e.g., HS256, RS256) and token type (JWT).
- Payload: Contains claims - statements about the user or resource, such as:
- Standard claims: Issuer (iss), expiration time (exp).
- Custom claims: Playback restrictions, user permissions, or resource-specific data.
- Signature: Generated by combining the encoded header, payload, and a secret key. This ensures the token’s integrity and prevents tampering.
How FastPix uses JWT
JWTs authorize access to protected resources, such as video streams. For example:
- A user requests playback of a private video using a playbackId.
- FastPix validates the JWT’s signature to ensure it was signed with the correct Signing Key and has not been altered.
- Claims (e.g., expiration time, permissions) are verified to confirm the token is valid and grants the required access.
This process ensures secure, efficient resource delivery while adhering to security standards.
How to generate JWT for secured playback Id
Step 1: Create a Signing Key
Signing keys are used to generate JWT signatures. To create one navigate to the Signing Keys section of your FastPix Dashboard or use our APIs.
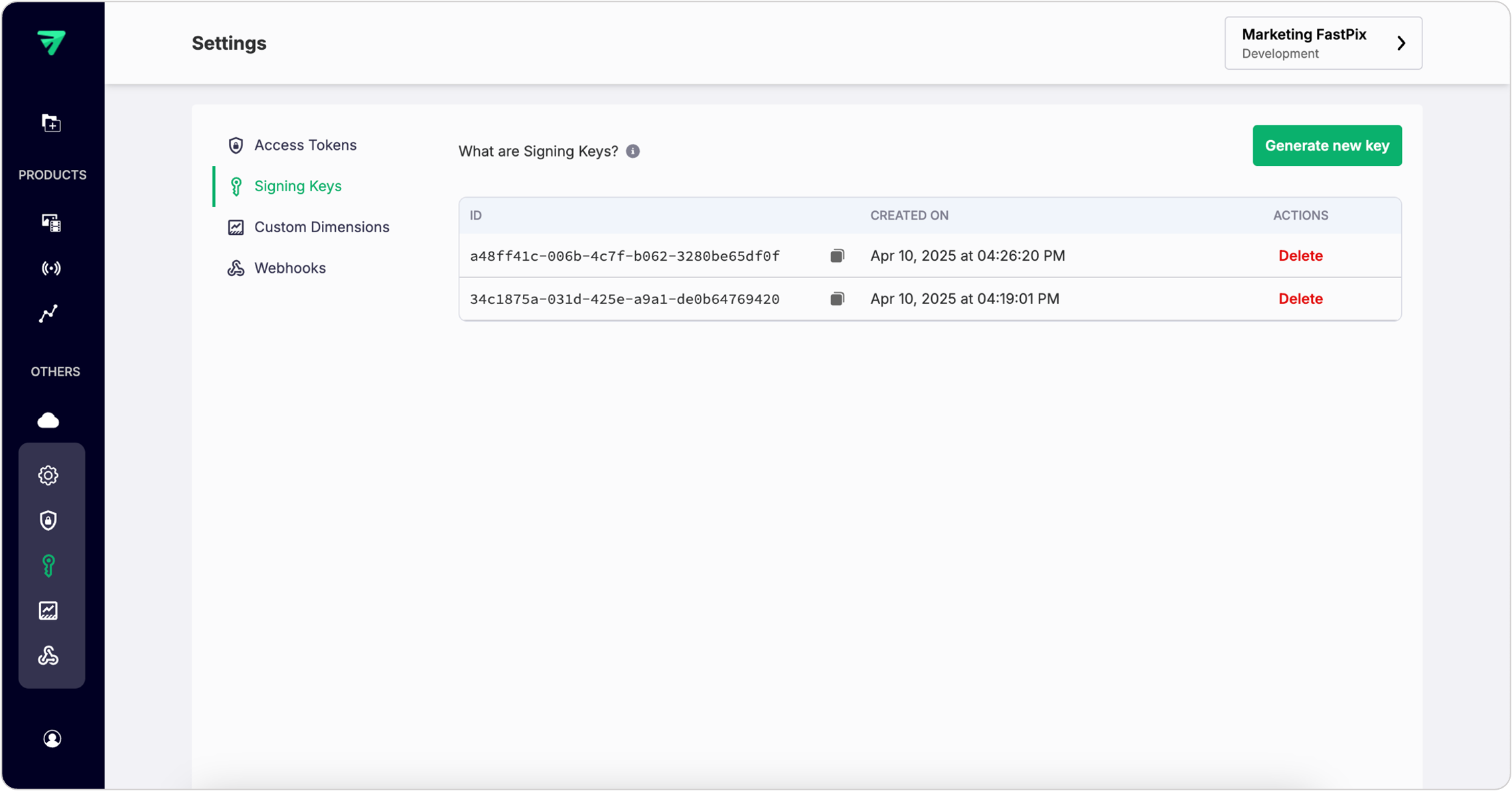
FastPix generates a new key pair (public/private keys) and a signing key Id.
- Store the generated private key and signing key Id securely - never expose it in client-side code or public repositories.
- The public key will be stored at FastPix used to verify tokens.
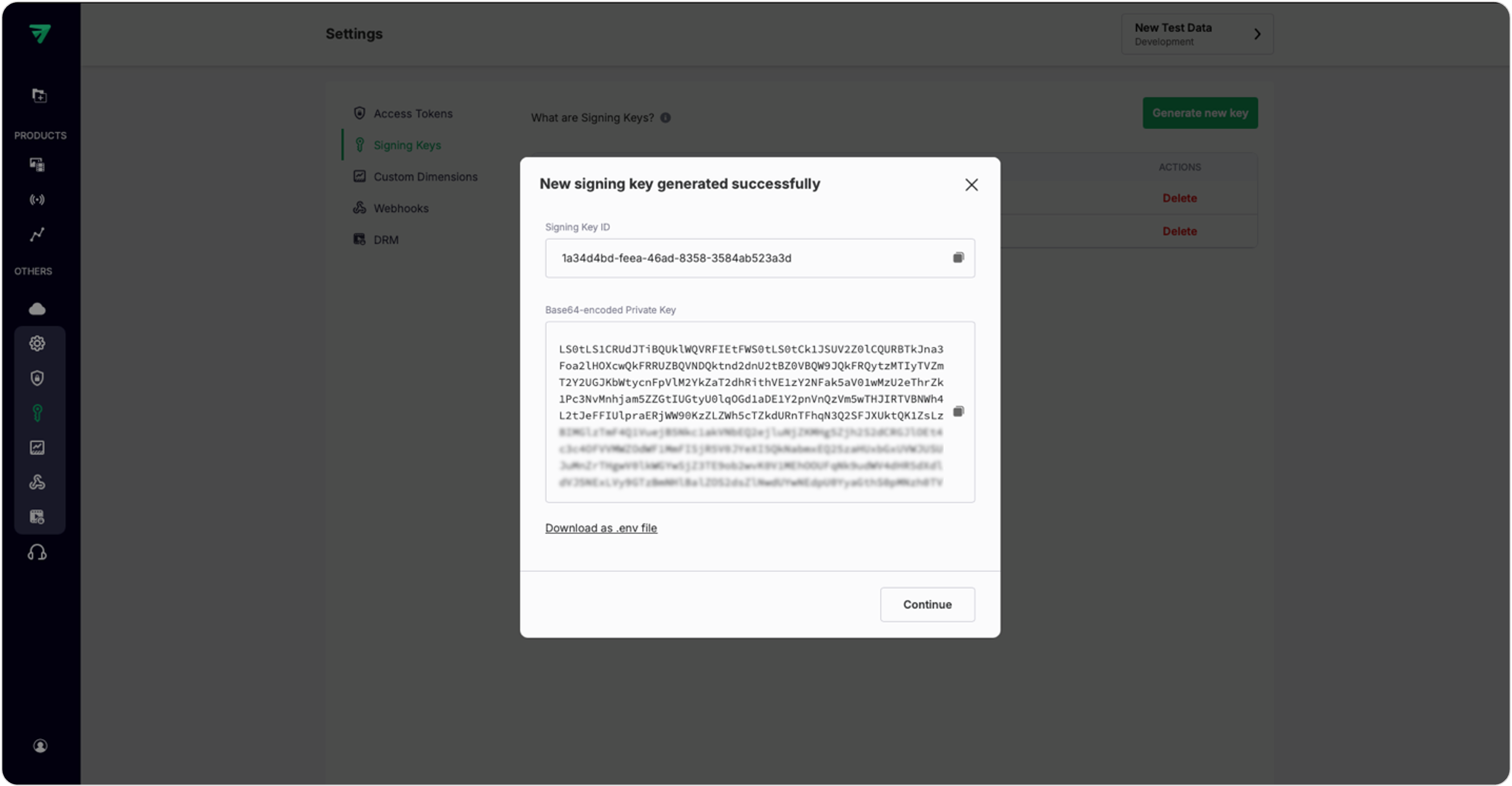
Using APIs to create signing keys
Refer the Create a signing key API reference.
You can create a new signing key pair for FastPix. When you call this endpoint, the API generates a 2048-bit RSA key pair. The privateKey
will be returned in the response, encoded in Base64 format, and you will receive a unique key id to reference the key in future operations.
{
"success": true,
"data": {
"id": "fc9d9368-6ee5-4b16-ae50-880a2374bdc4",
"privateKey": "LS0tLS1CRUdJTiBQUklWQVRFIEtFWS0tLS0tCk1JSUV2Z0lCQURBTkJna3Foa2lHOXcwQkFRRUZBQVNDQktnd2dnU2tBZ0VBQW9JQkFRREtaN1JKT1IrbXZGeVQxSWFIL0hVUkYwQnRncDJzK0srdUd4TUZ4N1JiaGNudVBMYU14WjM1b0lNWndhdHJrdDFDM3JxVFZsQzBsSnExeENFTyt3Zi9JNHQ0bktmUFB2WG83NGFCQi82YmR0MXpaSHp0OGFIenBnL3YrdEtCWVc5SEdWQ0tYc2JpNjczbHgwcFhHdXVnem8wdnZMR2lKWDBiL0Z4WEI5U1R3RkV5Q1dQOFJhczZ3VWVuSUdVM2UwMmJiV3Z4UnNoMWNER2xSRk0Ux4Uk5MTnhoMjM5UEdUT3BuWmdvTFp5Skt4b2REN1FpV1N1eDVZY1Z0MFgrSk9rZXNBOEpjM1ZtM0tGc0IwL3RYck9QQWdNQkFBRUNnZ0VBUHhNWUxLVmZocTgyVGw4eFdWbEVCZ0p2OG5COHdIVnpFZGVnRXZJTDgyVjY2d0lDaFZYa0IvR01TVStBSXZMT2Z0TTM0MGhIdUM2REU5ZTkwWlJMQnFoR0ExMFdNbEJWZzdSNC91YkY0aDZsbmhzWGozTDRYQnhJNVNrTnhvSGRrcE9COU16YVA4YmxFNkVLT3FES0F2KzdJY0EwdnVuZDFnWExwTmRzMkduTW5nZW1qOUhJZWh1eTNLY0taNHlheFo1YkRKVEpLZlFrTlFDQzhOR0hIdWovQmRWUnU1RHRrZVdNMFFpN2FKeW5lSXRxOGhtbXdxcVNMTnpTOTZtcHpBdzF3RzFXTHVML1A3TGxJekVWa2ZJUFc0SW1zRXhsSG5FUG0ySld1RUNMQ1pRL25NODdhQXVXekROektCYW51LzZhdXhnSDB2Wnc5YWowTmxNNFFRS0JnUURPM3Y0YlN4bWluZGJpVEdSaXdFVXVyODh4TVA3M2U5RSt2SHlzb3JZMWZycFpkdXJHOVlFb09MUFN1YnQ5WkhZNFhGOFhxRWZ4bE94d294eDVzcU9PcGNMTnFnOWhTSWxPaXEyYmVnN2V2RGpOMml6b3JKRFl3VEhGQ0Era25FbmFpL0J2TU16N3AyVVkrUEEzUnJ4Z25BK3RkQlErZWlSZ1c0WmhnMkhWcndLQmdRRDZlVEpwRTRxZVFYdmpnMy9FS081UkllRklZOHphTGMvMVVHODBqNmVvbStNK3UyTmdUVDJqVmNyMkdQbjZTbHRNRlJNem5qOVJHYmQ1MCt5a2k0Y1NYU1JPdE44alV2M0FseHJtZzEwVTVtSWIrUXFIZ3g2QldyeXkvakxHYXVvMUJnVFg1dDZ0VXVEUUZuVDJSM2xoNGRNZ044T3V4VlR3OCtadGloSllJUUtCZ1FERE00ZHpHWnBHNThrc0lBbFpaVFBpcWVKSCtJT2Q0eWUrbXZ6SnFzhXTVRWd293MzllUFhxdEhQOE9weCtxUmdaSWtxREhabzArRE5UL3JUUVM3Ty9leHpHT21QSXV3MjBmZ3VWU2NZWUxRbHgwVjdmajN5Q3JvRk1YYzZ2dW1XZHMrMFdQckg3bnFjb1R1NCtHZjZ4R0k1QVUvLzRRS0JnUUR6TFcvdjdIVU1xTzhyT0tSM1FuWCtkekpPSWZibGJNMFdrdjBrdnNROFF2MGlEclN3N3MwRkkycGwvR0hXeXhKUWo3V1F5L2NWT2k2VUxWajNlQyt2ZUphamc1K1FvQ2FWTVIrQTVkRWRWWCt6UU5za0xmMFVBWkJyQjdrc1F1a1lpYnR5RWtmblp5dTFXOWc2czdINWdsS0VXUiszTXdjQTJRdkRGZVl4Z1FLQmdDWVdlKzQ4bVVaUEl5ZnR4NVFaQllnYTE2blpndzYxZmxtdEdpQlVGWGVMR3BTaU1XNXc5R3RYVDZPbFh1Zy91TkNKbHR4TDE4c0NEeDNVaU9DNWFTMEN4OTc5TlFrSm1YRWw1UDNtMFNGaVU4VlZ0SFp1dHd3SWFKTFZockZ1T3NJV1BtRFN4aHhMaFpPNmJ5aWRwbHlXLzl1eGpwMlZrQ0Y3OGd5QXRRSWsKLS0tLS1FTkQgUFJJVkFURSBLRVktLS0tLQo=",
"createdAt": "2024-01-11T10:00:06.618993Z"
}
}
Step 2: Generate JWT with claims
Construct the JWT payload with claims required by FastPix. Common claims include:
- kid: The key ID of the signing key.
- aud: Audience (who or what the token is intended for)
- iss (Issuer): The issuer of the token. (e.g., "yourcompany.com").
- iat: Issued at (seconds since Unix epoch)
- exp (Expiration): Token expiry time (e.g., 1 hour from now).
{
"kid": "26338ada-fcf4-4434-b5b0-2ba77dde5d98",
"aud": "media:16af5b33-cf4d-4717-9b90-ce429a124455",
"iss": "fastpix.io",
"sub": "",
"iat": 1744780459,
"exp": 1744866859
}
Step 3: Signing JWT with generated signing key
Use a JWT library to encode and sign the token. Below is a generic example (language-agnostic):
-
Encode header:
Base64UrlEncode('{"alg":"HS256","typ":"JWT"}')
-
Encode payload:
Base64UrlEncode('{"kid":"26338ada-fcf4-4434-b5b0-2ba77dde5d98","aud":"media:16af5b33-cf4d-4717-9b90-ce429a124455","iss":"fastpix.io","iat":1744780459,"exp":1744866859}')
-
Generate signature:
HMACSHA256(base64UrlHeader + "." + base64UrlPayload, YOUR_SIGNING_KEY)
-
Combine all parts:
token = base64UrlHeader + "." + base64UrlPayload + "." + base64UrlSignature
Example:
const jwt = require('jsonwebtoken');
const token = jwt.sign(
{
iss: 'your-api-key',
exp: Math.floor(Date.now() / 1000) + 3600, // 1 hour
playbackId: 'video123'
},
'YOUR_SIGNING_KEY',
{ algorithm: 'HS256' }
);
Testing JWTs during development
To streamline JWT creation and validation, FastPix provides a user-friendly web based JWT Signer tool where you can input parameters, generate tokens, and validate them.
Access the tool: https://jwt.fastpix.app/
You can create JWTs by inputting your:
- Signing Key ID
- Playback ID
- Signing Key / Secret
The system will generate a secure JWT based on these parameters. Save this token for playback or validation workflows.
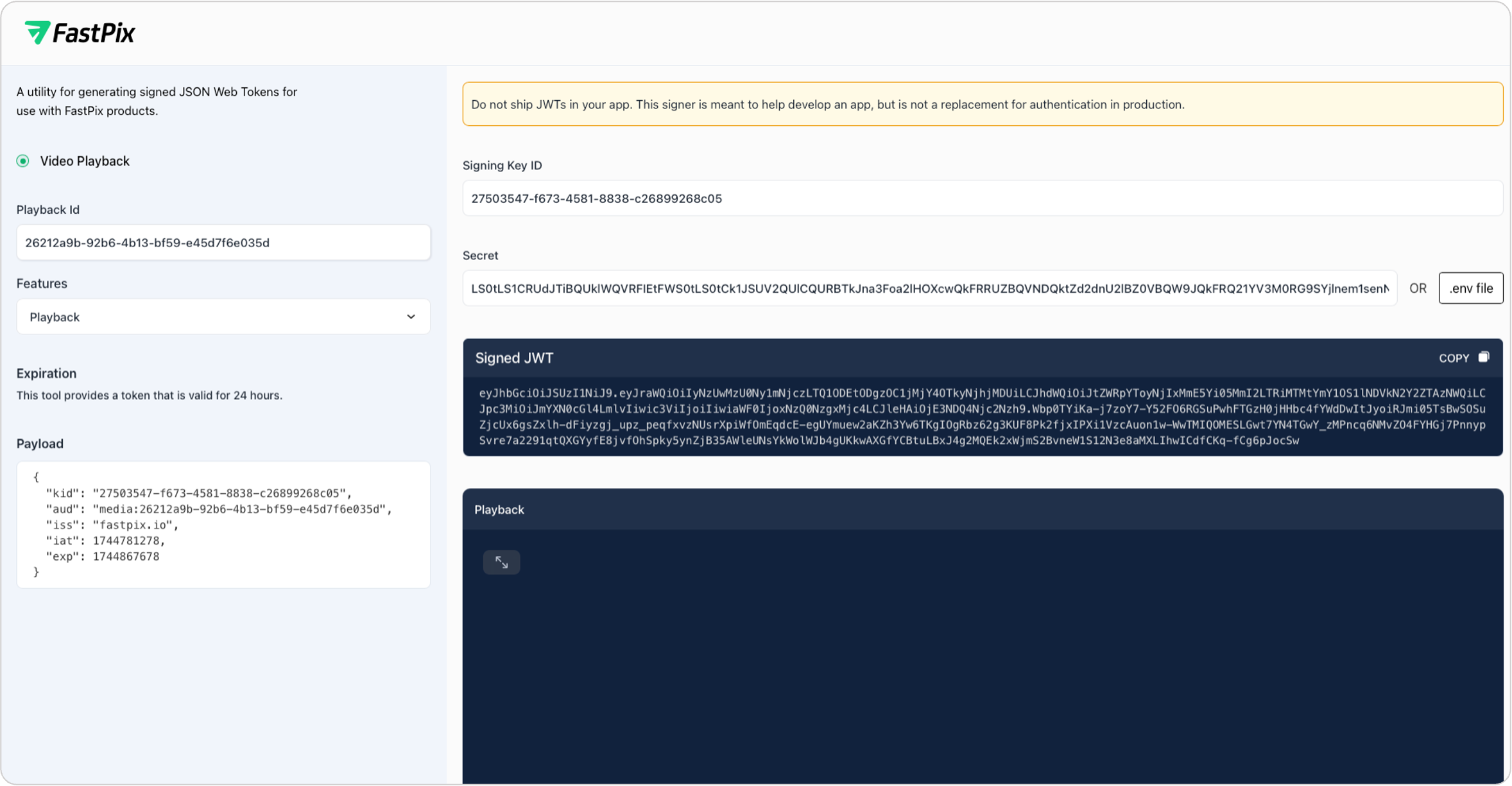
PLEASE NOTE
Do not ship JWTs in your application. This signer is meant to help develop an app, but is not a replacement for authentication in production.
Secured video playback with JWTs
Include the JSON Web Token (JWT) in your media stream URL using the token
query parameter. FastPix will inspect and validate the JWT to make sure the playback request is allowed.
https://stream.fastpix.io/{PLAYBACK_ID}.m3u8?token={JWT}
Validate your token integrity (Optional)
Before deploying tokens in production, use the JWT Decoder to:
- Paste your generated token.
- Verify its structure, claims (e.g., expiration time), and cryptographic signature.
- Ensure compliance with FastPix’s security requirements.
This step lets you crosscheck your tokens are correctly formatted, unexpired, and properly signed - preventing playback errors in live environments.
SECURITY NOTES
- Always sign tokens on a secure server.
- Rotate signing keys immediately if a private key is compromised.
- Use short-lived tokens to minimize risks from token leakage.
Updated about 1 month ago